Nonlinear Quantum Optics Group
Our group explores how we can create strong optical nonlinearity in a medium at light levels of individual photons. Such a quantum nonlinear medium mediates an effective interaction between single photons and thus opens the possibility of manipulating light at its most fundamental level. Our goal is to realize a system that enables full control over the quantum state of few photon light pulses.
Latest news
© nqo
New Bachelor’s & Master’s thesis topics now online1
New Bachelor’s & Master’s thesis topics for the WS25/26 are online – take a look now!
Julia wins Poster Award at ECAMP152
We're happy to share that Julia brought home the Poster Presentation Award at ECAMP15 in Innsbruck!
DPG news spread around the world3
In the UN’s "International Year of Quantum Science and Technology," Sebastian shared insights on quantum computing.
© Nqo
Trip to Phantasialand4
Our research group had a fun and exciting trip to Phantasialand in Brühl!
Our research
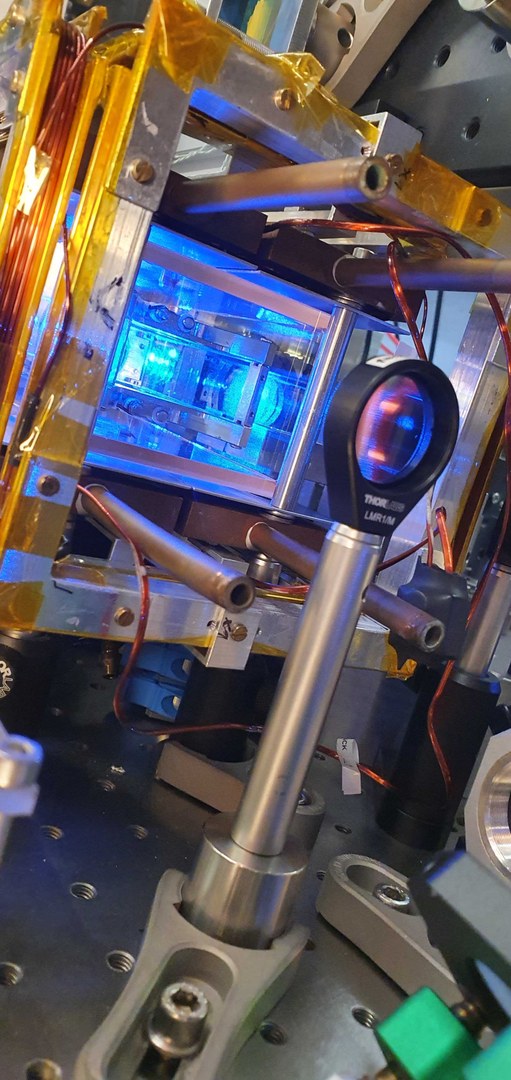
© nqo
Rubidium Rydberg Nonlinear Quantum Optics
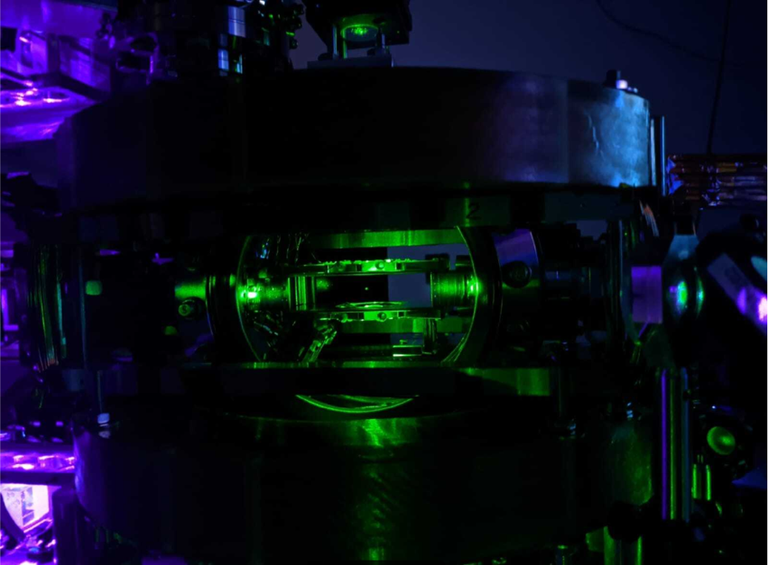
© nqo
Ytterbium Rydberg Nonlinear Quantum Optics

© nqo
Fiber Cavity Optomechanics
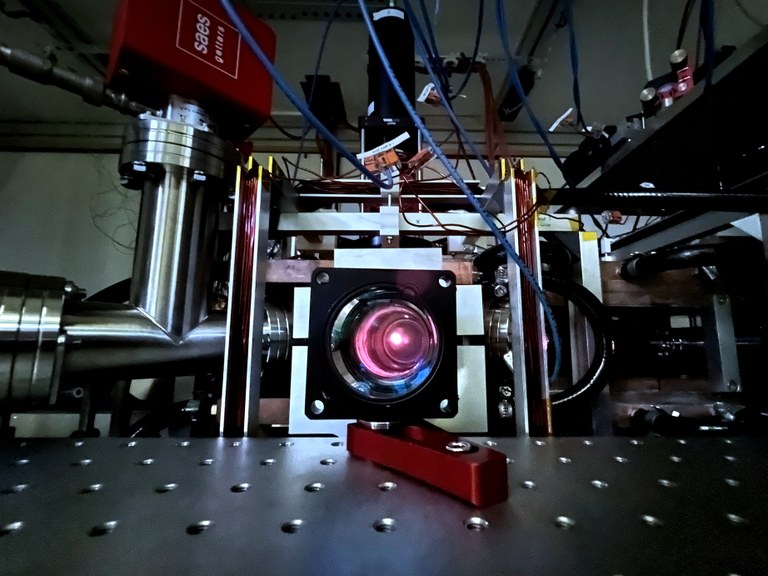
© nqo
Hybrid quantum optics
Get in touch!
Prof. Dr. Sebastian Hofferberth
hofferberth@iap.uni-bonn.de
Tel.: +49 (0)228 733477
Contact the institute
Institut für Angewandte Physik
Wegelerstraße 8
Universität Bonn
53115 Bonn, Germany
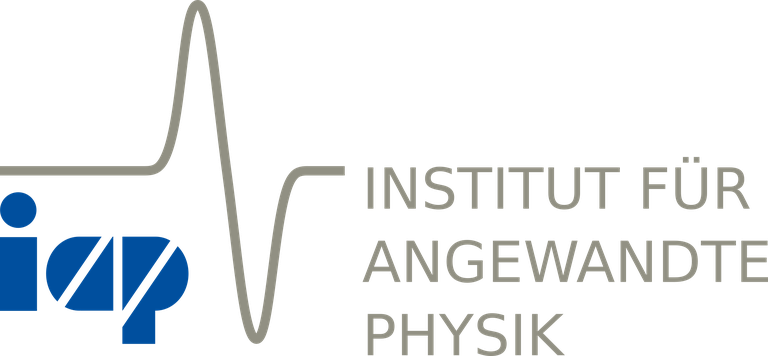
© IAP
Links
- https://www.iap.uni-bonn.de/nqo/en/nqonews/new-bachelors-masters-thesis-topics-now-online
- https://www.iap.uni-bonn.de/nqo/en/nqonews/julia-wins-poster-award-at-ecamp15
- https://www.iap.uni-bonn.de/nqo/en/nqonews/dpg-news-spread-around-the-world
- https://www.iap.uni-bonn.de/nqo/en/nqonews/trip-to-phantasialand
- https://www.iap.uni-bonn.de/nqo/en/nqonews/latest-news
- https://www.iap.uni-bonn.de/nqo/en/research/rubidium-rydberg-nqo
- https://www.iap.uni-bonn.de/nqo/en/research/ytterbium-rydberg-nqo
- https://www.iap.uni-bonn.de/nqo/en/research/fiber-cavity-optomechanics
- https://www.iap.uni-bonn.de/nqo/en/research/hybrid-quantum-optics
- http://iap.uni-bonn.de